Today I changed my site from WordPress to a static one. The loading speed should improve much.
I checked it with Google PageSpeed Insights, and do more optimization according to the result.
In this post, I will explain what is inline and differing JavaScript/CSS, and how to use it to improve your page loading speed.
External files referenced in the page: JavaScript, CSS, etc. often block the browser from rendering the page.
Front-end performance tuning must eliminate any potential rendering blocking points to allow the browser to render the entire page in the shortest amount of time possible.
JavaScript
Let’s have a look at this simple HTML:
|
In the above code, when the browser is parsing the script
tag, since the browser doesn’t know what page.js
will do to the page, the browser needs to stop rendering, download and execute page.js
and then continue rendering the rest of the page. Any delay in downloading page.js
will also affect the rendering of the entire page.
So, how to avoid it?
Inline JavaScript
If the initial rendering of the page does rely on page.js, we can use inline JavaScript.
|
Delayed loading
If the initial rendering of the page does not depend on page.js
, we could delay the loading of page.js
and load it after the initial content of the page is rendered.
|
Async/Defer loading
There is another two script attribute called async and defer, which allow us to reduce the time for downloading the scripts.
In this case, the browser will render the rest of the page as it downloads page.js
.
|
There are trivial differences between them, async will pause HTML parser to execute the JS when downloading finished.
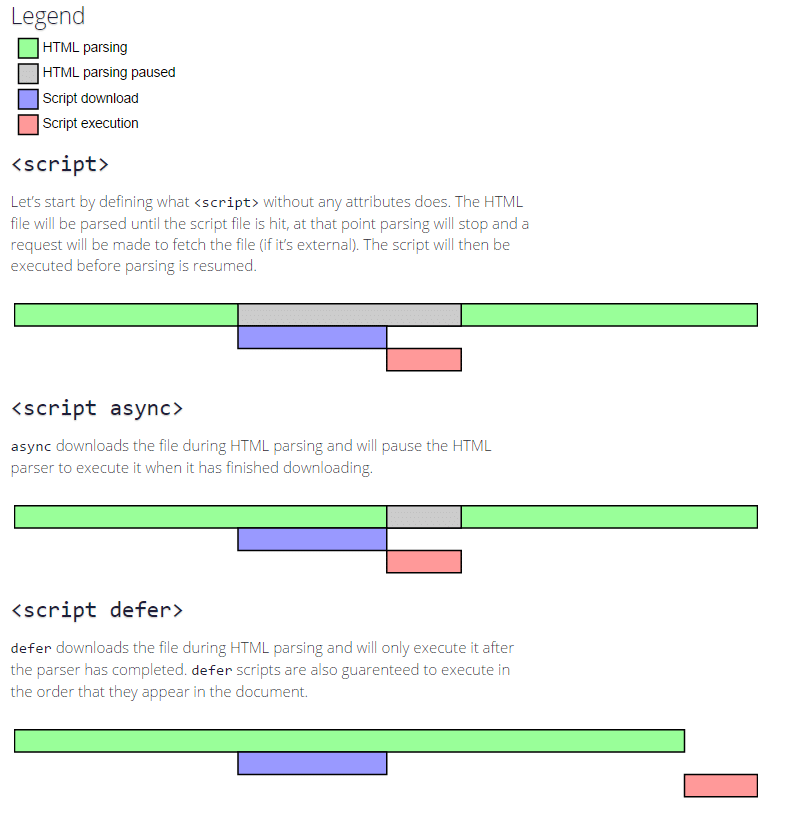
CSS
Since CSS determines the style and layout of DOM elements, the browser will wait for the CSS file to load and parse before rendering the page when it encounters it.
Inline CSS
We can add Inline CSS to the CSS code needed for those page first screen renderings.
|
Delay loading of CSS
For CSS that is not needed for first screen rendering, we can still use the file form and load it after the page content is rendered.
|
Conclusion
The JS and CSS required for the initial rendering of the page can be inserted directly into the <head>
tag in code form. All external file references can be placed after the page content, and JS files can also be loaded asynchronously.
Join my Email List for more insights, It's Free!😋